Passport-SteemConnect
A SteemConnect authentication strategy for Passport.
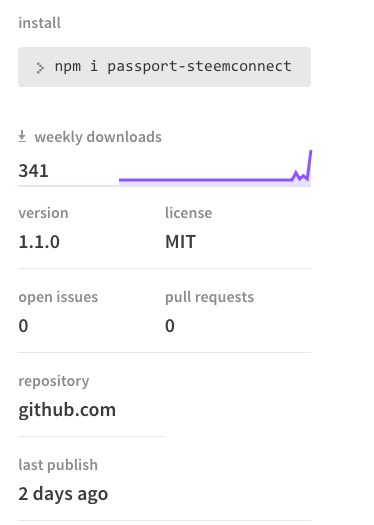
var mongoose = require("mongoose"),
passportLocalMongoose = require("passport-local-mongoose")
var UserSchema = new mongoose.Schema({
_id: Number,
username: String,
password: String,
accessToken: String,
refreshToken: String,
profile: Object
});
UserSchema.plugin(passportLocalMongoose);
module.exports = mongoose.model("User", UserSchema);
passport.use(
new Strategy(
{
authorizationURL: `https://steemconnect.com/oauth2/authorize`,
tokenURL: `https://steemconnect.com/api/oauth2/token`,
clientID: process.env.clientID,
clientSecret: process.env.clientSecret,
callbackURL: `http://localhost:3000/auth/oauth/oauth2/callback`,
scope: ["vote", "comment"]
},
function(accessToken, refreshToken, profile, cb) {
let userInfo = {
_id: profile.id,
username: profile.username,
password: null,
accessToken: accessToken,
refreshToken: refreshToken,
profile: profile
};
User.update({ _id: userInfo._id }, userInfo, { upsert: true }, function(
err,
result
) {
err ? console.log(err) : cb(null, profile);
});
}
)
);
passport.serializeUser(function(user, cb) {
cb(null, user.id);
});
passport.deserializeUser(function(obj, cb) {
User.findOne({ _id: obj }, (err, user) => {
err ? console.log(err) : cb(null, user.profile);
});
});
/*
mongoose.connect("mongodb://localhost/db");
*/
This module lets you authenticate using SteemConnect in your Node.js applications.
By plugging into Passport, SteemConnect authentication can be easily and
unobtrusively integrated into any application or framework that supports
Connect-style middleware, including
Express and NextAuth
To use this passport Strategy just install passport-steemconnect
Install
$ npm i passport-steemconnect --save
Passport Strategy: https://github.com/SMWARREN/Passport-SteemConnect
Passport Strategy With Example : https://github.com/SMWARREN/SteemConnect-Next-Auth-Micro-Service
Congratulations @mildfun! You received a personal award!
You can view your badges on your Steem Board and compare to others on the Steem Ranking
Vote for @Steemitboard as a witness to get one more award and increased upvotes!