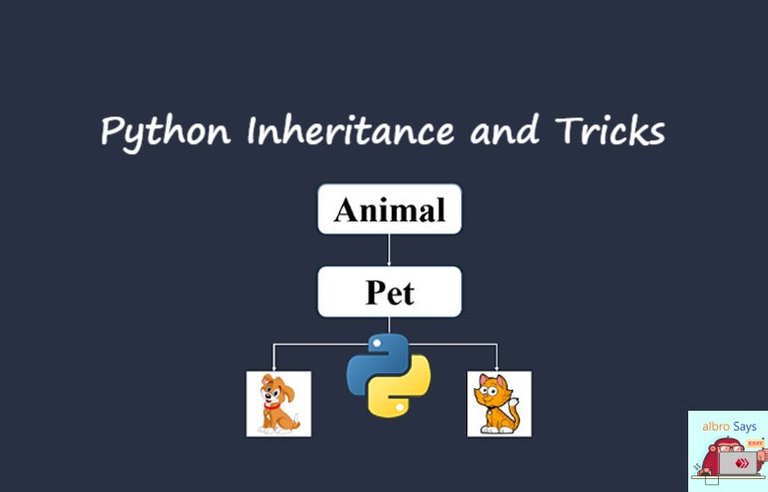
With the help of inheritance in Python, we can inherit the properties and behaviors of a class from a higher class. For example, the cat class inherits from the animal class so that we don't have to redefine all functions. In this post, I'll talk about inheritance in Python.
In the principles of object-oriented programming, we have a basic discussion called inheritance in classes. With the help of inheritance, the properties and behaviors of a class are inherited from a higher class.
Just as in human reproduction, children inherit properties and behaviors from their parents. Of course, each child can have their own unique properties and behaviors.
To better match inheritance in Python to the real world, I'll give another small example. Suppose we have a class called "Pet
" which is our pet.
In this case, we can have subclasses like cat
and dog
. Both of them are a type of pet that, in addition to having the properties of a pet, also have unique properties and behaviors.
Inheritance in Python
We are all familiar with class definition in Python. To make a class inherit from another class, we use parentheses in front of the class name.
Suppose we have two classes A
and B
. We want B
to inherit from A
, that is, to have all its properties and methods in itself. For this, we will have a structure similar to the following:
class A:
pass # class A body
class B(A):
pass # class B body
An example of Python inheritance
Let's go back to the last example I gave. First, we consider a class called Pet
for a pet in Python. In the constructor of this class, we define the name, color and age of the pet. We also have two methods:
- The
getName()
method returns its name. - The
makeSound()
method generally prints an irrelevant text. It is clear that the sound of a cat and a dog is different. Later we will learn to redefine these methods for each class.
class Pet:
def __init__(self, name, color, age):
self.name = name
self.color = color
self.age = age
def getName(self):
return self.name
def makeSound(self):
print("bla bla bla")
In very simple language, a method is a function that is defined in a class and will be available through the objects of that class.
Now I define the Cat
class. I also define a simple method to determine if the cat is chasing the rat:
class Cat(Pet):
def chasingRat(self):
print("It's chasing rat!")
Now I make an instance (object) of cat:
kitty = Cat("Kitty", "white", 2)
With the help of the IDE, I check what methods and properties I have access to on this object:
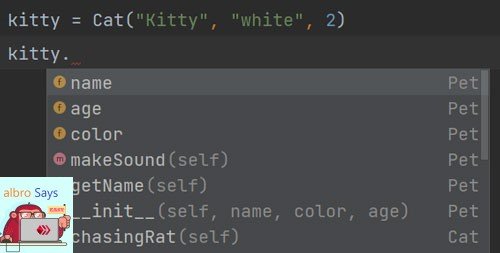
As you can see, I can use the methods and properties of both the Pet
class and the Cat
class. This means inheritance in Python! 🙂
If you pay attention, the constructor of the parent class was also used when creating the cat. In the following code, I access its various methods:
print(kitty.getName()) # Kitty
kitty.chasingRat() # It's chasing rat!
Rewrite in child class
When we want to rewrite a method of the parent class in the child class, it is called Method Overriding. I want to override the makeSound()
method in the Cat
class and change its body.
class Cat(Pet):
def chasingRat(self):
print("It's chasing rat!")
def makeSound(self):
print("meowwww!")
Now if we create an object from the Cat
class and call makeSound()
, the method inside the Cat class will be executed. In this way, we were able to rewrite the method during inheritance in Python.
kitty = Cat("Kitty", "white", 2)
kitty.makeSound()
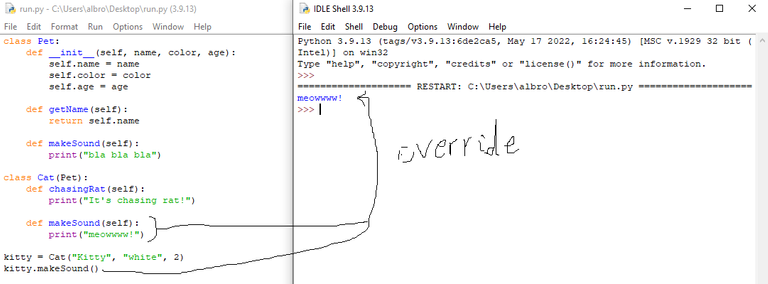
Important points of inheritance in Python
You see that we were able to inherit between two classes so easily. It is better to review 2 very important points:
Multiple inheritance in Python
Python supports multiple inheritance. It means that each class can inherit from two or more other classes at the same time.
For multiple inheritance in Python, it is enough to separate the names of the parent classes with a comma (,
) and do the same as before:
class A:
# body
class B:
# body
class C(A, B):
# body of C that inherited from A, B
Note that the order of these classes is important. If there are several methods with the same name in these classes, the method on the rightmost side of the class will be overridden.
Hierarchical inheritance
You've probably guessed by now that we can have sequential inheritance in Python classes. If we take the original example of the post, the pet itself inherits from the Animal
class. Therefore, we can have a structure similar to the following.
class Animal:
pass
class Pet(Animal):
pass
class Cat(Pet):
pass
In order not to make the codes long, I've removed the body of the classes and used the pass
keyword.
Parent class in Python inheritance
Sometimes it is necessary to access the methods of the parent class in the child class. We have two methods for this:
- Using the
super()
function to access the parent class - Using the name of the parent class and accessing its methods
Let's say I want to get the cat's eye color in the Cat
constructor as well. For this I can rewrite the whole body; It means to re-initialize all the variables. I can also get help from the Pet
constructor, similar to the following code:
class Pet:
def __init__(self, name, color, age):
self.name = name
self.color = color
self.age = age
class Cat(Pet):
def __init__(self, name, color, age, eye_color):
super().__init__(name, color, age)
self.eye_color = eye_color
kitty = Cat("Kitty", "white", 2, "black")
If we want to do the second method, the child class will change to the following state. Note that in this method, we have to give self
as an input argument to the method we called.
class Cat(Pet):
def __init__(self, name, color, age, eye_color):
Pet.__init__(self, name, color, age)
self.eye_color = eye_color
Suggestion: If the class only inherits from one class, use super()
and in multiple inheritance in Python, use the names of the classes.
👍
!LUV
https://leofinance.io/threads/albro/re-leothreads-dyahbe6u
The rewards earned on this comment will go directly to the people ( albro ) sharing the post on LeoThreads,LikeTu,dBuzz.
Congratulations @albro! You have completed the following achievement on the Hive blockchain And have been rewarded with New badge(s)
Your next target is to reach 200 upvotes.
You can view your badges on your board and compare yourself to others in the Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
To support your work, I also upvoted your post!
Congratulations!
✅ Good job. Your post has been appreciated and has received support from CHESS BROTHERS ♔ 💪
♟ We invite you to use our hashtag #chessbrothers and learn more about us.
♟♟ You can also reach us on our Discord server and promote your posts there.
♟♟♟ Consider joining our curation trail so we work as a team and you get rewards automatically.
♞♟ Check out our @chessbrotherspro account to learn about the curation process carried out daily by our team.
🥇 If you want to earn profits with your HP delegation and support our project, we invite you to join the Master Investor plan. Here you can learn how to do it.
Kindly
The CHESS BROTHERS team
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.