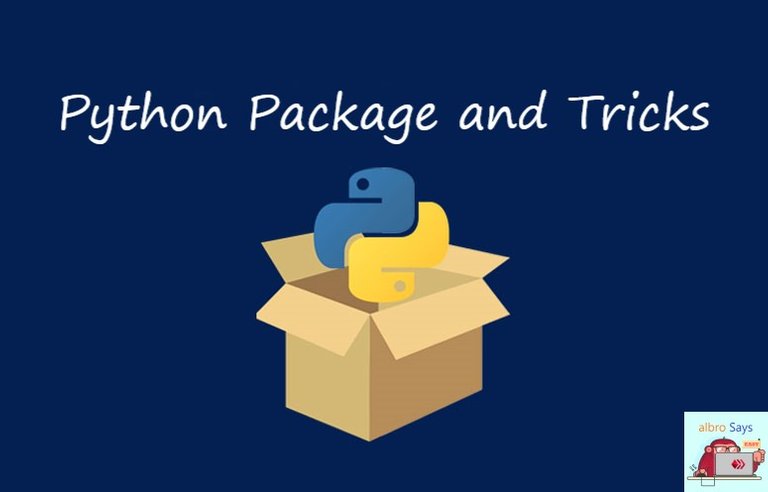
A package in Python is a collection of modules! When we put several modules in a folder, we say that we have made a package in Python. This is done for better code management and organization. In this post, I'm talking about the packages and its tricks.
In order to understand all the explanations in this post, it is better to be familiar with modules. If you're not familiar, see the post related to modules in Python.
A module was the file of our codes that we used in other codes. Usually, each module has a specific purpose. This type of code classification helps us to use our modules in other projects in addition to easy change management.
Python packages
In very simple language, a package in Python (Python Package) is a number of modules that are placed in a folder! Usually, the modules that are included in a package have a similar purpose. For example, the authentication part of a program or user management can be a package.
I'll proceed with a simple example for better understanding.
Suppose we have two modules for entering and exiting users. To keep the codes simple, each has just one function to print a specific message.
# login.py
def do_login():
print("logging in ...")
# logout.py
def do_logout():
print("Logging out ...")
I put the login.py
and logout.py
files in a folder called auth
.
Creating a package in Python
In order to tell Python that this folder isn't a normal folder and is a package, we need a file called __init__.py
next to these files.
This file can be empty and contain no code. When we call one or more modules of this package in our program, first the __init__
file is executed and then the module codes are entered in the program.
Therefore, you may want to define special functions, variables or classes in this file according to your needs.
Python package call
Now I'll create a file named app.py
next to the folder to use the package we made; So my file structure so far looks something like the following tree:
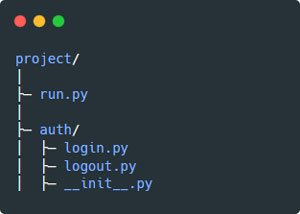
Similar to calling modules, we can call and use package modules with the import
statement. The name of the package folder is placed first and then the name of the module. The two are separated by a dot (.
).
# app.py
import auth.login
auth.login.do_login()
In the following code, I have used the from ... import
statement to use one or more modules:
from auth import login, logout
login.do_login()
logout.do_logout()
In this simple way, we were able to create a simple package with two modules and use it.

We all categorize the files we have on our computers or mobile phones in folders and subfolders. In Python packaging, we can also take help from the hierarchical structure of folders.
It means that our package doesn't need to have a folder containing several modules; Rather, each package can have subpackages.
Here I've created some different files and packages next to my Python code so that we can check how to call them together.
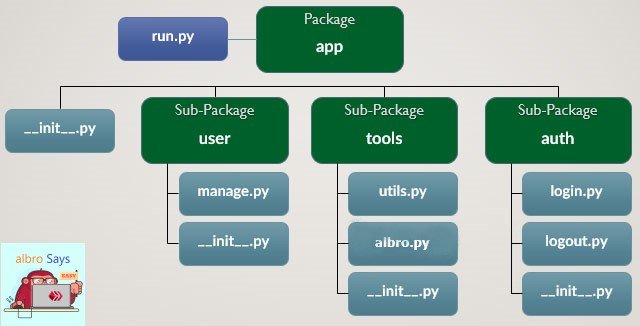
Pay attention that we create __init__.py
file in all folders.
Similar to what we had so far, we can directly import
packages into the program:
import app.auth.login
# usage:
app.auth.login.do_login()
To simplify the use of functions, classes and variables within each module, it may be better to use the from import
structure.
In this way, when calling the functions, it is enough to put the name of the module at the beginning:
from app.auth import login
login.do_login()
If you want to use only one function, class or variable inside the module, direct import
of the same one is also a good method.
from app.auth.login import do_login
do_login()
I suggest you use this mode only when you are going to import
one or two items from the package. If you enter a large number with full namespace
(the same mode without writing the package name), the complexity of your code will increase.
Modules in a Python package
You've probably guessed by now that we can also use aliases for modules or items we import
. For example, in the following code, I've called the login
module from the app.auth
package with the alias lgn
in my Python code:
from app.auth import login as lgn
lgn.do_login()
Of course, it is better to use less abbreviations whose meanings are difficult to understand. For example, here lgn
was only for training and it is better not to use it in the real project.
If you remember, the utils module we created contained a class and a list of names in addition to a function.
In the following code of this module, I call my app.tools
package and use the list and class.
from app.tools import utils
print(utils.users)
p1 = utils.Person("xeldal")
You can see that we have a lot of freedom in defining Python package module codes. So whenever you feel your code needs more organization, you can use modules and packaging in Python.
I hope you are familiar with how to define a package in Python and how to use it. If you have any questions or doubts about this, the comments section is for you.
Another one good article 😎
https://leofinance.io/threads/albro/re-leothreads-cfofagmg
The rewards earned on this comment will go directly to the people ( albro ) sharing the post on LeoThreads,LikeTu,dBuzz.
Yay! 🤗
Your content has been boosted with Ecency Points, by @albro.
Use Ecency daily to boost your growth on platform!
Support Ecency
Vote for new Proposal
Delegate HP and earn more
Congratulations!
✅ Good job. Your post has been appreciated and has received support from CHESS BROTHERS ♔ 💪
♟ We invite you to use our hashtag #chessbrothers and learn more about us.
♟♟ You can also reach us on our Discord server and promote your posts there.
♟♟♟ Consider joining our curation trail so we work as a team and you get rewards automatically.
♞♟ Check out our @chessbrotherspro account to learn about the curation process carried out daily by our team.
🥇 If you want to earn profits with your HP delegation and support our project, we invite you to join the Master Investor plan. Here you can learn how to do it.
Kindly
The CHESS BROTHERS team
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.