It is very important to test for bugs and incorrect behaviors on any project before shipping them to production in order to ensure that the app will function just as expected and to protect it from unwanted behaviors during any update.
It's also noticed that Using testing tools to automate tests can save time, especially when working on projects with a large codebase.
One of the best practices for testing your application is to prioritize which parts to test. For example:
- Test high-value features (i.e., the main features of your app)
- Test edge cases in high-value features (e.g., testing to see if the features written in high-value features can be broken)
For example, in a lottery application, I worked on as a frontend developer, I was asked to add a feature to limit users from purchasing more than 50 tickets at a time. Testing this feature would be considered an edge-case in a high-value feature.
- Test for things that are easy to break
- React component testing
- User interaction with the DOM
- Conditional rendering
- Default or custom hooks
I have several projects with large codebases that need to be tested, but since I am new to testing JavaScript code using testing techniques, I have decided to start with testing my portfolio code because it has a smaller codebase. In my portfolio, I have implemented a logic to fetch my Hive posts and display them on the front end. This will be a good starting point for me.
There are many unit testing frameworks available to choose from, such as;
- Jest
- Mocha
- Jasmine. etc
However, my portfolio website was built using Next.js, and testing Next.js apps is slightly different from testing vanilla JavaScript. Here is a list of testing tools that can be used for testing Next.js codebases
- Cypress
- Playwright
- Jest and React Testing Library
- Vitest
I decided to use Cypress for testing because I watched a video about it and I thought it was cool. I installed it by running npm install --save-dev cypress
, which installs it in my development environment as we don't need it in production.
Then I included the cypress script inside of my package.json file so that Cypress would run the tests from the command line.
"cypress:open": "cypress open",
"cypress:run": "cypress run"
}
Next, I ran npm run cypress:open
then it opened a new window as seen below.
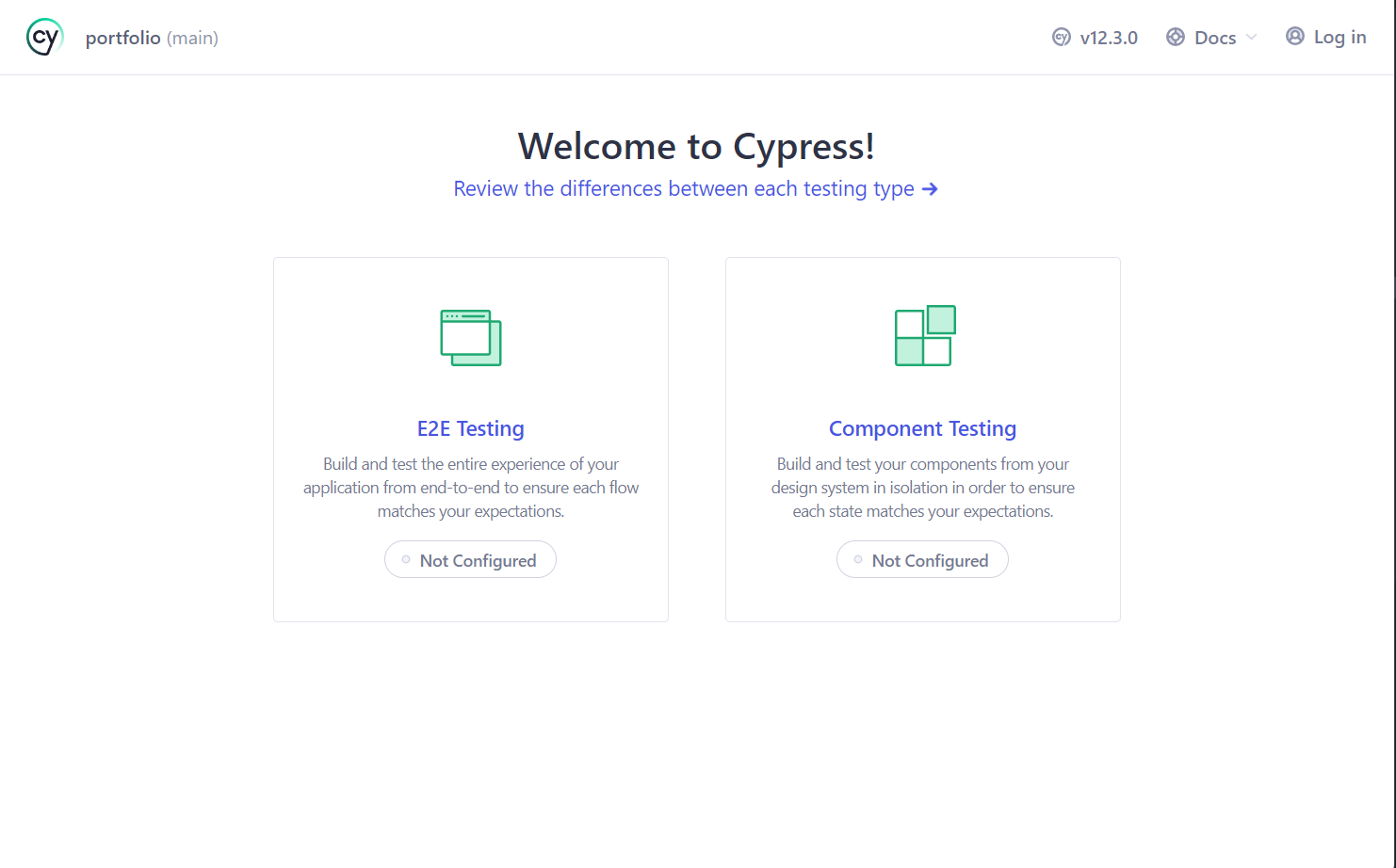
There are two ways to test apps with this tool. Component & End-To-End.
I decided to use E2E testing, which required me to create a new test. As I will be testing my portfolio code and the Hive posts it fetches, I created a blog.cy.js file and opened it with VSCode.
Before writing any code for the test, I inspected my blog section to understand what to test for. In my case, I had written JavaScript code to fetch the three most recent posts from my Hive account.
const getUserPost = async () => {
const post = [];
hivejs.api.getDiscussionsByBlog({ tag: 'rufans', limit: 3 }, (err, data) => {
data.map((postDetails) => {
post.push(postDetails);
setPosts(post);
});
});
};
Therefore, I decided to write a test to check if the number of posts fetched is equal to 3. To do this, I inspected the HTML element that holds my posts, which was the element. Then, I wrote a test code for Cypress to do the following:
- Visit my base url at /blog, as all pages in Nextjs are automatically compiled as routes.
- Get the element and verify that it has a total length of 3.
The code looked something like this;
describe('should fetch only 3 post', () => {
it('should fetch 3 latest blog posts', () => {
cy.visit('http://localhost:3000/blog')
cy.get('a').should('have.length', 3)
})
})
And here's the result showing that it passed the test
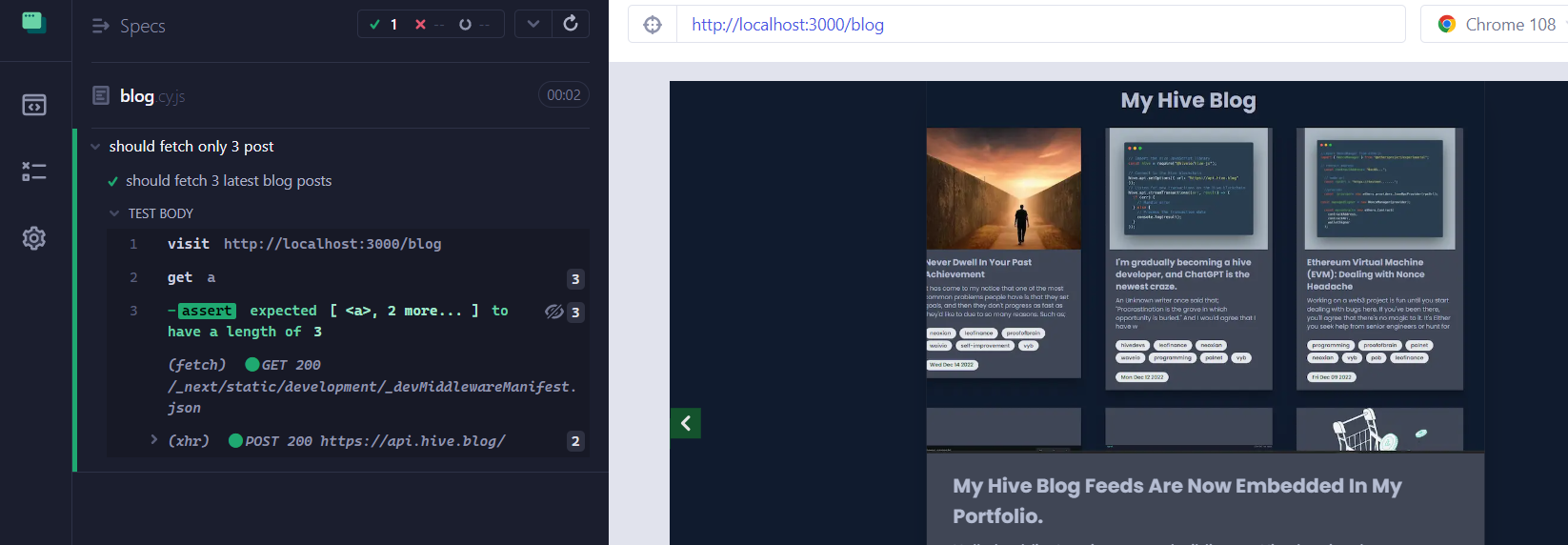
What if I change the number of posts to fetch? You'll notice that it will fail. Let's try it;
const getUserPost = async () => {
const post = [];
hivejs.api.getDiscussionsByBlog({ tag: 'rufans', limit: 10 }, (err, data) => {
data.map((postDetails) => {
post.push(postDetails);
setPosts(post);
});
});
};
The failing result;
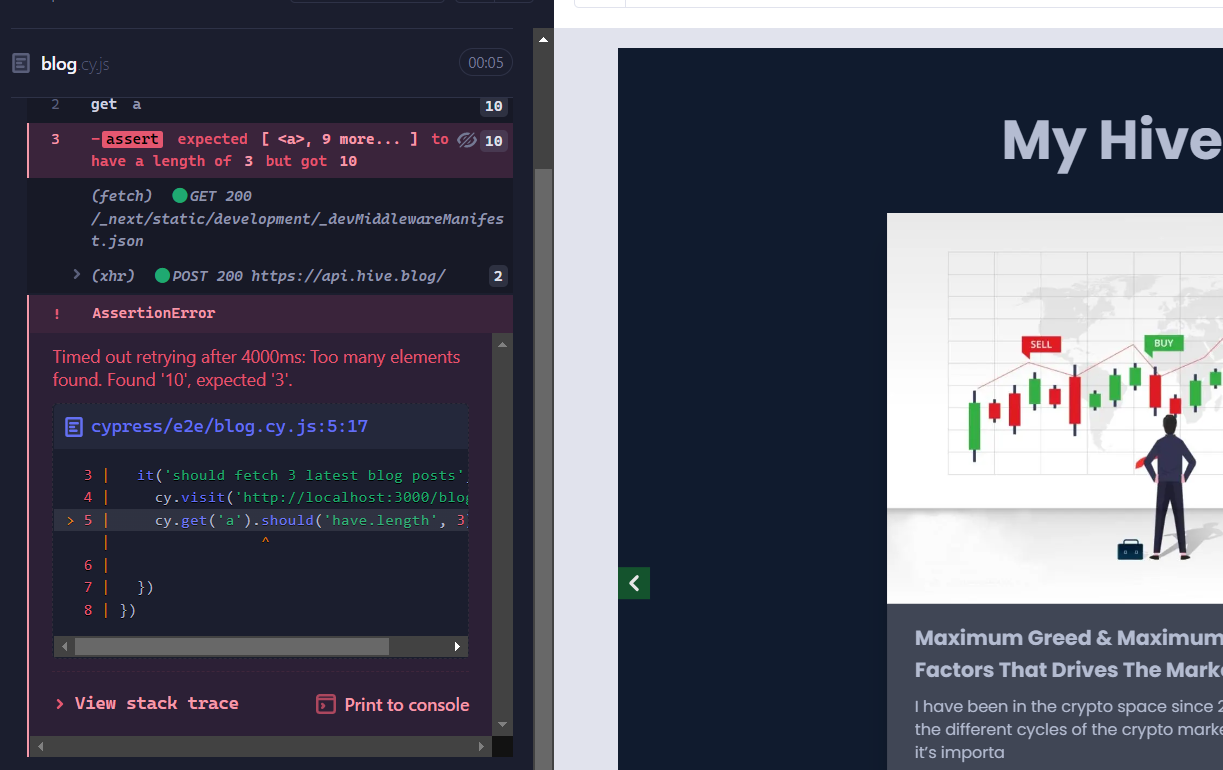
That's it! I am very much new to using this testing tool. This is just the tip of the iceberg when it comes to unit testing I hope to do more complex tests soon.
The rewards earned on this comment will go directly to the people sharing the post on Twitter as long as they are registered with @poshtoken. Sign up at https://hiveposh.com.
@tipu curate
Upvoted 👌 (Mana: 0/48) Liquid rewards.
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.