Many interesting things happens on hive right now, we have hard fork of hived, new version of hivemind coming soon, wrapped hive was just released, a lot of changes in leo, and new games are created. As a newcomer it can be little overwhelming, so i decided to create a series of articles for those who wants to engage in hive from programming perspective, and start from the basics.
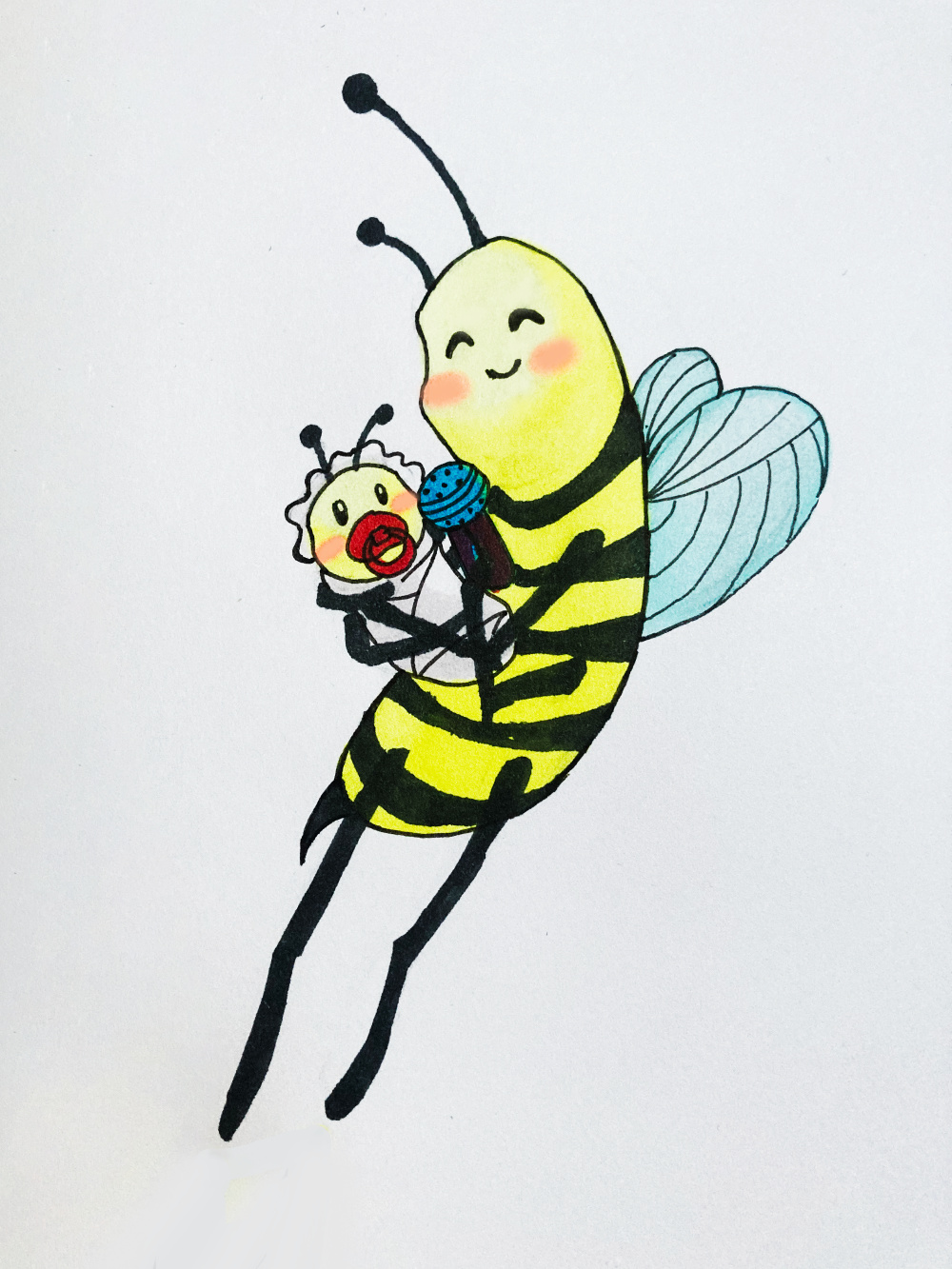
graphic made by @thenoy
The easiest option to create new account is just by visit on website https://signup.hive.io/ and choosing one of the account providers. But if we want to have more control on process of creating keys, or automate process we can do some scripting to achieve that.
Requirements:
- Hive account with at least 3 HIVE to pay for new account
We will use beem – a python library to interact with hive. We need to install it at first based on: https://beem.readthedocs.io/en/latest/installation.html
There is also nice introduction to beem so I recommend to read it.
Generating Keys
First we need to generate master key, it’s random value which together with account name can generate our account keys. It is also used to recover keys if we lose them, so it's important to write it down and put in safe place. To do this we need to write in command line:
beempy keygen
We should get results like this:
+------------+-------------------------------------------------------+
| Key | Value |
+------------+-------------------------------------------------------+
| Public Key | STM7TGdernF9y9VaabsyUyF3fVibttc5MLJrUGj1YodiYk4vv23Tf |
+------------+-------------------------------------------------------+
+------------------+----------------------------------------------------------------------------------------------------------------------------------------------------------+
| Key | Value |
+------------------+----------------------------------------------------------------------------------------------------------------------------------------------------------+
| Account sequence | 0 |
| Key sequence | 0 |
| Key role | owner |
| path | m/48'/13'/0'/0'/0' |
| BIP39 wordlist | match hybrid asthma jump piano gentle alley approve inspire prize door post grass matter awful festival shed infant vibrant erupt wet ignore giant stand |
| Private Key | 5KX... |
+------------------+----------------------------------------------------------------------------------------------------------------------------------------------------------+
now we can use our private key to generate keys used in hive blockchain with command:
beempy keygen --import-password -a account.name
where account.name is the name of account we want to create, command line asks about our Master Key, so we paste Private Key generated in beempy keygen
command
result should look like this:
+--------------------+-------------------------------------------------------+
| Key | Value |
+--------------------+-------------------------------------------------------+
| Username | account.name |
| owner Public Key | STM8Guc4yxWPbSrXEg746u2BqCpZHX9aGKPujyPH586KTpzj2pM4s |
| active Public Key | STM6Ljc7Nqc6rTYeQHM2P1u4DQBJRcXdMd59fqwoekChMYQSaemEN |
| posting Public Key | STM6yAP1x5QVSU6icYH5ukH82MvdwrRGwWeHmP4gbffcauuy554mi |
| memo Public Key | STM54JkiS5zmAj1BpMTBG8rJod2EmNp7LQPjpygyhtviriz7nDgZM |
+--------------------+-------------------------------------------------------+
+--------------------------+-----------------------------------------------------+
| Key | Value |
+--------------------------+-----------------------------------------------------+
| Username | account.name |
| owner Private Key | 5JT... |
| active Private Key | 5JE... |
| posting Private Key | 5Ke... |
| memo Private Key | 5KS... |
| Backup (Master) Password | 5KX... |
+--------------------------+-----------------------------------------------------+
If we want make account extra secure, it can be generated on offline computer where we install beem, and then use only needed keys, so owner and master password never touches online hardware
More details about types of keys can be found here: https://wallet.hive.blog/@yourusername/permissions
Ok, we have all the keys, now we need to create and broadcast transaction on the blockchain. To do that we can create a simple script in .py file and run it:
from pprint import pprint
from beem import Hive
from beem.account import Account
from beem.instance import set_shared_blockchain_instance
wif = "CREATOR_ACTIVE_KEY"
creator_name = "CREATOR_NAME"
new_account_name = "NEW_ACCOUNT_NAME"
new_master_password = "NEW_MASTER_PASSWORD"
hive = Hive(nobroadcast=True)
hive.wallet.wipe(True)
hive.wallet.unlock("wallet-passphrase")
hive.wallet.addPrivateKey(wif)
set_shared_blockchain_instance(hive)
# Our account used to create new account
account = Account(creator_name, blockchain_instance=hive)
pprint(hive.create_account(
account_name=new_account_name,
creator=creator_name,
password=new_master_password
))
We should replace values "CREATOR_ACTIVE_KEY", "CREATOR_NAME", "NEW_ACCOUNT_NAME", "NEW_MASTER_PASSWORD" with our data and run the script. As we have Hive(nobroadcast=True) that it's only generating and not broadcasting transaction, we can safely preview if everything is correct.
We should get result similar to:
/usr/bin/python3.6 "/var/www/hive/account creator/create.py"
{'expiration': '2020-09-06T16:56:16',
'extensions': [],
'operations': [['account_create',
{'active': {'account_auths': [],
'key_auths': [['STM6Ljc7Nqc6rTYeQHM2P1u4DQBJRcXdMd59fqwoekChMYQSaemEN',
'1']],
'weight_threshold': 1},
'creator': 'nalesnik',
'fee': '3.000 HIVE',
'json_metadata': '',
'memo_key': 'STM54JkiS5zmAj1BpMTBG8rJod2EmNp7LQPjpygyhtviriz7nDgZM',
'new_account_name': 'account.name',
'owner': {'account_auths': [],
'key_auths': [['STM8Guc4yxWPbSrXEg746u2BqCpZHX9aGKPujyPH586KTpzj2pM4s',
'1']],
'weight_threshold': 1},
'posting': {'account_auths': [],
'key_auths': [['STM6yAP1x5QVSU6icYH5ukH82MvdwrRGwWeHmP4gbffcauuy554mi',
'1']],
'weight_threshold': 1}}]],
'ref_block_num': 41437,
'ref_block_prefix': 1051053763,
'signatures': ['206e45e8fb70e58cd2ae7150699b2b33f69e3a702e017d902f514f6082da8edd9e74c5e9e6b6a5194fea89053a9a3ecb8443de6a987ef0e6ed5ccdd152994bbc02'],
'trx_id': '77e602db42af871dc78090e12c022651a0e9cf4c'}
Process finished with exit code 0
Now we verify, if public keys, are the same as generated from beempy. If everything is correct we can remove nobroadcast=True
and run script again to broadcast transaction and... BUM newborn bee is created :)
Extra options
There is a possibility to create account without paying 3 HIVE fee, but we need to have decent amount of Resource Credits, details can be found here: https://developers.hive.io/tutorials-recipes/account-creation-process.html
Why multiple accounts?
Having multiple accounts can be useful, if we write posts on multpile fields its good to separate them. For example one account for posts about programming, one for hobby interests etc. Also if we want to create bot account to automate interactions with blockchain, its also useful to run it on separate account.
Bonus
I created account named grab10hive
with master password: nalesnik
. I transfer 10 HIVE to it. Feel free to transfer funds to your account if they are still there.
If you have any questions feel free to ask in comments.
I wouldn't separate content to different accounts, nowadays that can be managed by communities (by default, what you post within communities is not re-blogged to your account's feed), so one can please their poetry audience and skydiving at the same time. Except for the case that you have different stage names (fragile-joe & racinggoose) ;-)
Of course, for development purposes you often need more than one :-)
I wonder when there will be someone to grab the reward.
Congratulations @nalesnik! You received a personal badge!
Participate in the next Power Up Day and try to power-up more HIVE to get a bigger Power-Bee.
May the Hive Power be with you!
You can view your badges on your board and compare yourself to others in the Ranking
Do not miss the last post from @hivebuzz:
Congratulations @nalesnik! You have completed the following achievement on the Hive blockchain and have been rewarded with new badge(s) :
You can view your badges on your board and compare yourself to others in the Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
Congratulations @nalesnik! You received a personal badge!
Wait until the end of Power Up Day to find out the size of your Power-Bee.
May the Hive Power be with you!
You can view your badges on your board and compare yourself to others in the Ranking
Do not miss the last post from @hivebuzz: